Spring 注解 @After,@Around,@Before 的执行顺序
概述
在Spring框架中,面向切面编程(AOP)是一种强大的技术,它允许开发者定义横切关注点,如日志记录、事务管理等,并将这些关注点从业务逻辑中分离出来。Spring AOP支持多种通知类型,通过注解来实现,其中@Before
、@After
和@Around
是最常用的三种。本文旨在详细解释这三种通知类型的执行顺序及其应用场景,帮助开发者更好地理解和应用Spring AOP。
准备工作
首先,确保你的项目已配置Spring AOP支持。这通常意味着你已经包含了Spring相关的依赖,并且开启了AspectJ的自动代理。
Maven依赖示例
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
配置类
确保Spring配置启用了AOP代理(如果使用Java配置):
@Configuration
@EnableAspectJAutoProxy
public class AppConfig {
}
示例代码
目标服务类
@Service
public class MyService {
public void doSomething() {
System.out.println("Executing: doSomething");
}
}
切面类
@Aspect
@Component
public class MyAspect {
@Before("execution(* com.example.service.MyService.doSomething(..))")
public void beforeAdvice() {
System.out.println("@Before: Logging before method execution.");
}
@After("execution(* com.example.service.MyService.doSomething(..))")
public void afterAdvice() {
System.out.println("@After: Logging after method execution.");
}
@Around("execution(* com.example.service.MyService.doSomething(..))")
public Object aroundAdvice(ProceedingJoinPoint joinPoint) throws Throwable {
System.out.println("@Around: Before method execution.");
Object result = joinPoint.proceed(); // Continue to the intercepted method.
System.out.println("@Around: After method execution.");
return result;
}
}
执行结果分析
当调用MyService
的doSomething
方法时,控制台输出如下:
@Around: Before method execution.
@Before: Logging before method execution.
Executing: doSomething
@Around: After method execution.
@After: Logging after method execution.
@Before
@Before
注解用于定义前置通知,表示在目标方法执行之前,切面中的代码会被执行。它不关心目标方法的执行结果,仅用于在真正执行业务逻辑之前进行一些预处理操作。
@After
@After
注解用于定义后置通知,表示无论目标方法是否正常执行完毕(即使发生异常),切面中的代码都会被执行。它通常用于资源清理或记录方法执行的最终状态。
@Around
@Around
注解提供了最强大的通知类型,它可以控制目标方法的执行。一个围绕通知可以决定是否执行目标方法,以及在方法执行前后执行自定义的行为。这使得@Around
能够同时实现@Before
和@After
的功能,并且可以对方法的结果进行操作。
总结
从上述代码执行结果中,我们可以清晰地看到@Around
、@Before
、@After
注解的执行顺序,当一个方法被多个切面的这些注解所影响时,它们的执行顺序遵循以下原则:
-
@Around:首先执行。如果有多个
@Around
通知,它们按照切面定义的顺序执行。每个@Around
都有机会决定是否继续执行链中的下一个通知或目标方法。 -
@Before:在目标方法执行之前,按照切面的定义顺序依次执行。
-
目标方法:在所有前置通知执行完毕后执行。
-
@After:无论目标方法是否成功执行或抛出异常,都会执行。执行顺序仍然遵循切面定义的顺序。
Spring AOP通过@Before
、@After
和@Around
注解为开发者提供了灵活的切面编程能力。理解它们的执行顺序对于正确地设计和实施切面逻辑至关重要。简而言之,@Around
通知最先有机会介入,接着是@Before
通知,目标方法随后执行,最后是@After
通知进行收尾工作。合理利用这些通知类型,可以有效地解耦横切关注点与核心业务逻辑,提高代码的可维护性和扩展性。
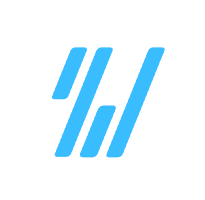
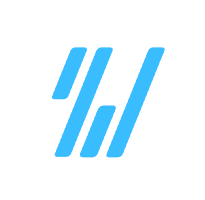
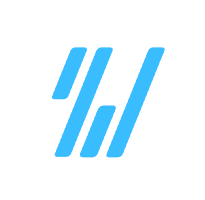
评论区(0)